堆排序
2024年9月2日大约 2 分钟
堆
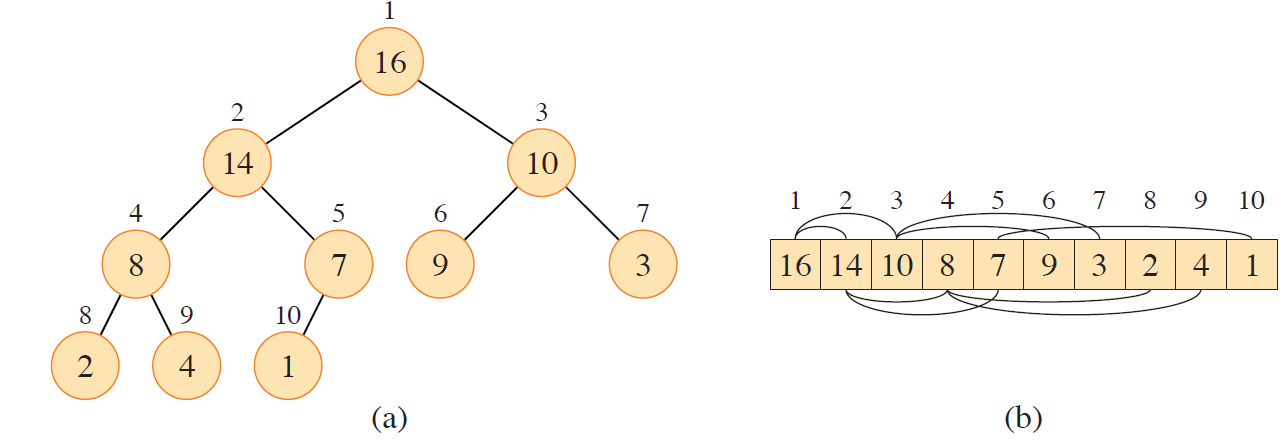
PARENT(i)
return i/2
LEFT(i)
return 2i
RIGHT(i)
return 2i+1
维持堆的性质
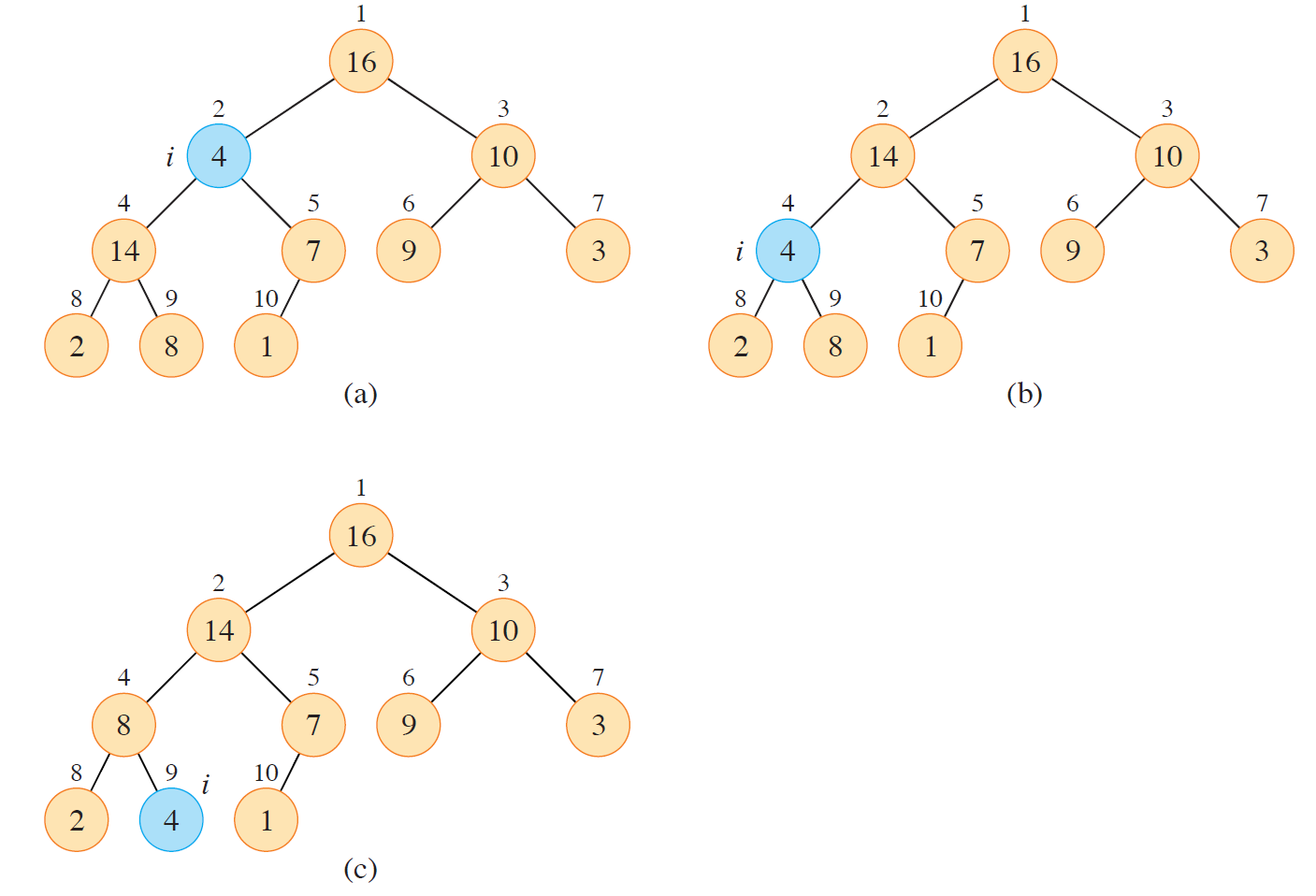
max_heapify(A,i)
l = LEFT(i)
r = RIGHT(i)
if l ≤ A.heap-size and A[l] > A[i]
largest = l
else largest = i
if r ≤ A.heap-size and A[r] > A[largest]
largest = r
if largest ≠ i
exchange A[i] with A[largest]
max_heapify(A,largest)
时间复杂度为 T(n) ≤ T(2n/3)+Θ(1) 根据主方法定理第二种情况可得 T(n) = O(lgn)
构造堆
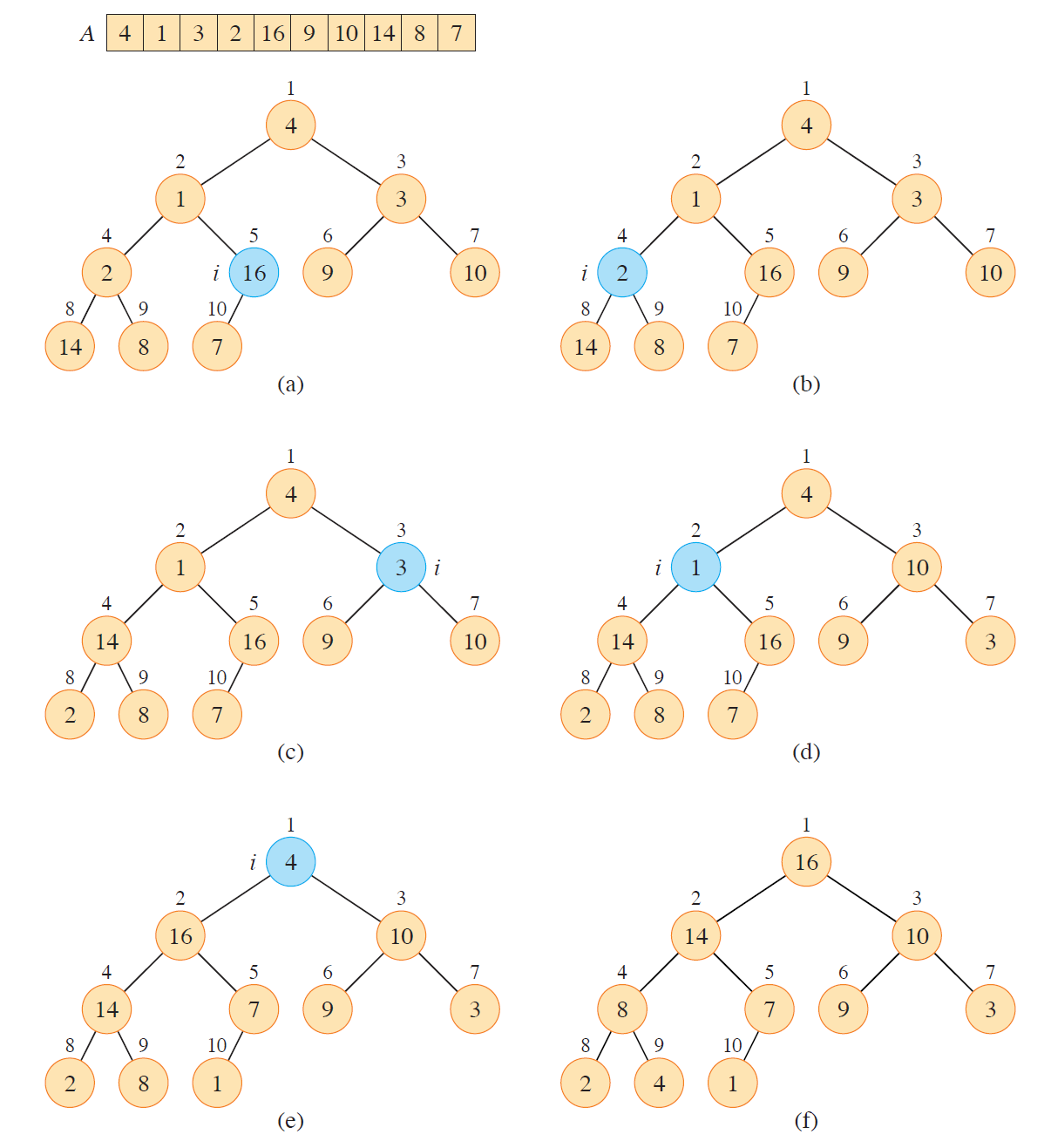
build_max_heap(A,n)
A.heap-size = n
for i = n/2 downto 1
max_heapify(A,i)
时间复杂度
含有 n 个元素的堆的高度 ,并且在任一高度 h 最多有 个结点,则有
堆排序
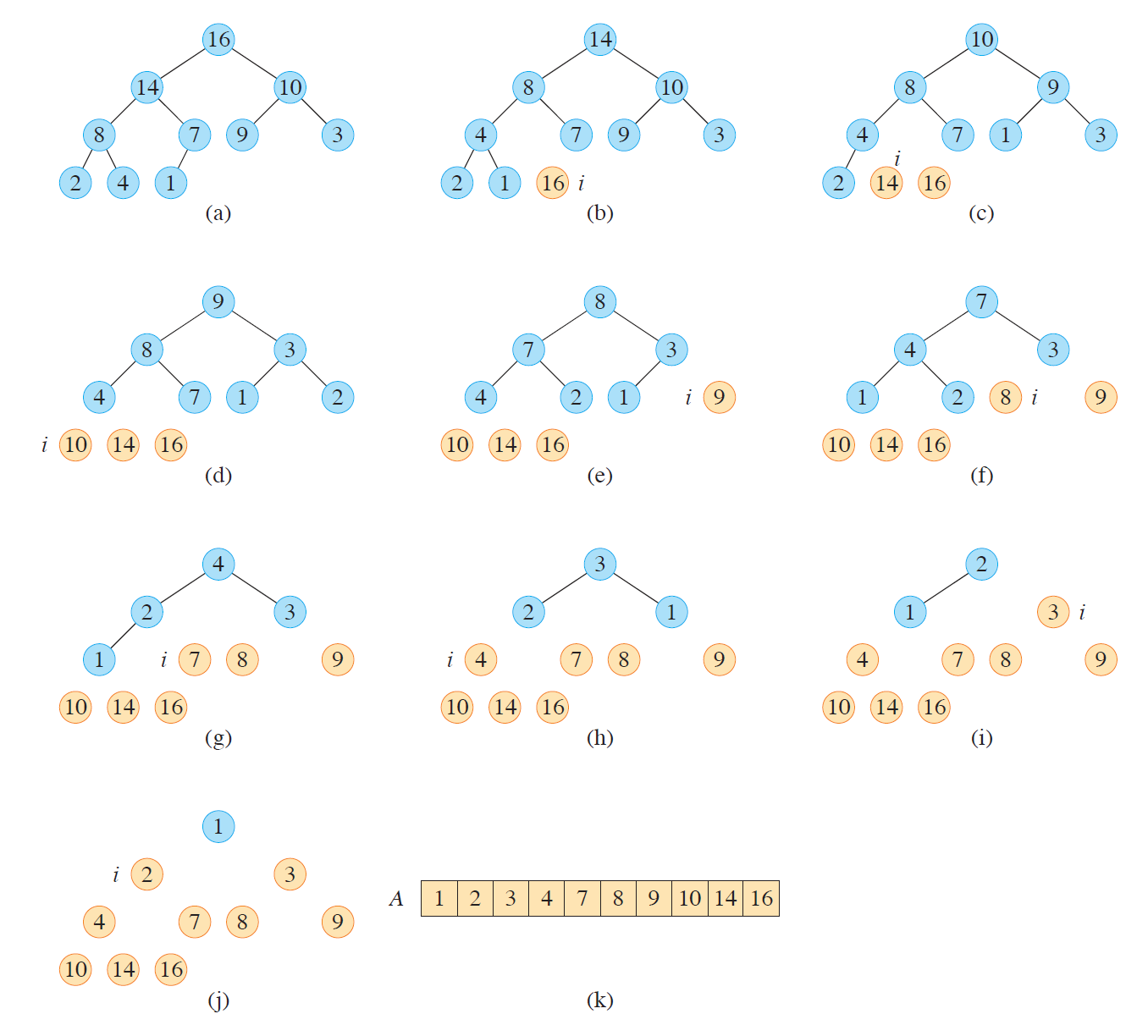
heapsort(A,n)
build_max_heap(A,n)
for i = n downto 2
exchange A[1] with A[i]
A.heap-size = A.heap-size - 1
max_heapify(A,1)
时间复杂度为 O(nlgn)
优先级队列
max_heap_maximum(A)
if A.heap-size < 1
return "heap underflow"
return A[1]
max_heap_extract_max(A)
max = max_heap_maximum(A)
A[1] = A[A.heap-size]
A.heap-size = A.heap-size - 1
max_heapify(A,1)
return max
max_heap_increase_key(A,x,k)
if k < x.key
error "new key is smaller than current key"
x.key = k
// find the index i where A[i] = x
while i > 1 and A[PARENT(i)].key < A[i].key
exchange A[i] with A[PARENT(i)]
i = PARENT(i)
max_heap_insert(A,x,n)
if A.heap-size == n
error "heap overflow"
A.heap-size = A.heap-size + 1
k = x.key
x.key = -∞
A[A.heap-size] = x
max_heap_increase_key(A,x,k)